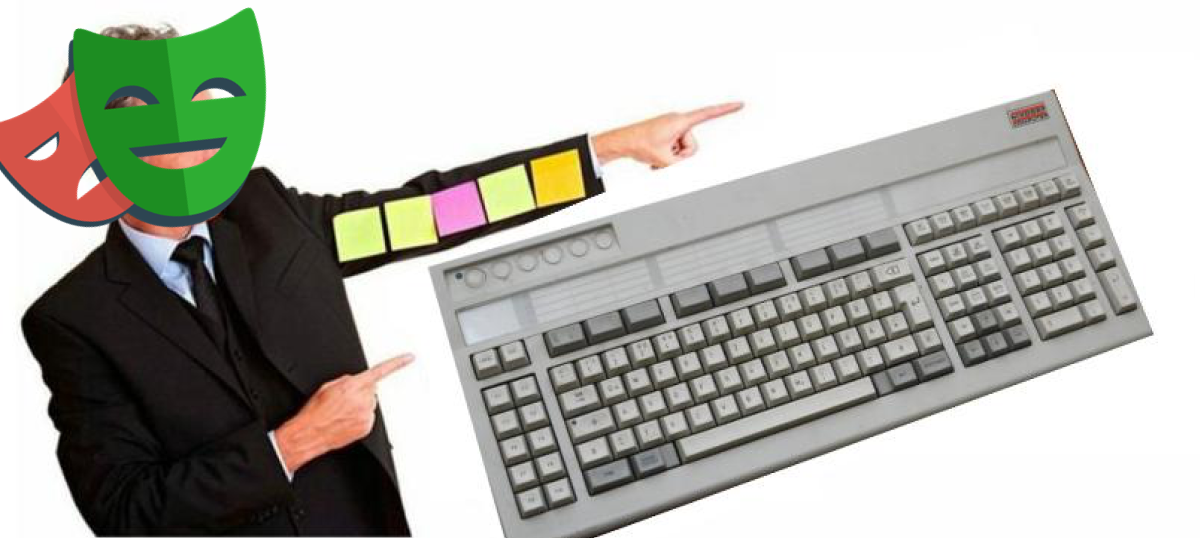
Form filling, text input, and hotkey pressing are crucial parts of user interface (UI) test automation. In this guide, we will study how to use keyboard events using Playwright.
Keyboard.press()
To simply press any keyboard key you can use the page.keyboard.press() method. It only executes keyDown and keyUp events:
await page.keyboard.press('A');
You can also add the timeout to wait between keyDown and keyUp in milliseconds adding the delay option:
await page.keyboard.press('A', {delay:1000});
Playwright supports not only the characters but also the modifiers and functional keys like Enter or Arrow:
await page.keyboard.press('Enter');
To avoid typos in big projects, I suggest storing all keys in one place:
export const KEYBOARD_KEY = {
A: 'a',
ALT: 'Alt',
ARROW_DOWN: 'ArrowDown',
B: 'b',
C: 'c',
CONTROL: 'Control',
D: 'd',
// ...
};
await page.keyboard.press(KEYBOARD_KEY.ARROW_DOWN);
Keyboard.press() shortcuts
Of course, you can use key combinations, for example, to copy-paste something. There are two ways to do that.
Use the keyboard.down() and the keyboard.up() methods combination to hold the Control button, then press the C button, and then release the Control button:
export const KEYBOARD_KEY = {
A: 'a',
ALT: 'Alt',
ARROW_DOWN: 'ArrowDown',
B: 'b',
C: 'c',
CONTROL: 'Control',
D: 'd',
// ...
};
await page.keyboard.down(KEYBOARD_KEY.CONTROL);
await page.keyboard.press(KEYBOARD_KEY.C);
await page.keyboard.up(KEYBOARD_KEY.CONTROL);
Or just use the KEY+KEY combination.
export const KEYBOARD_KEY = {
A: 'a',
ALT: 'Alt',
ARROW_DOWN: 'ArrowDown',
B: 'b',
C: 'c',
CONTROL: 'Control',
D: 'd',
// ...
};
await page.keyboard.press(`${KEYBOARD_KEY.CONTROL}+${KEYBOARD_KEY.C}`);
It is my favorite common approach to use keyboard shortcuts.
Learn all key values for keyboard events here.
Multiplatform keyboard.press() shortcuts
One of the common problems I faced at the beginning of my automation journey was the fact that some keys in MacOS and Windows/Linux are different. For example, there is no Control key on MacOS because there is a Meta (CMD or Command) key instead. It was a problem because my tests run on both Windows and MacOS platforms.
await page.keyboard.press('Control+C'); //Windows or Linux
await page.keyboard.press('Meta+C'); //MacOS
Fortunately, there is a pretty simple solution to handle it. The only thing you need is to detect the platform. In JavaScript, you can do this using an os.platform() method:
const os = require('os');
const platform = os.platform();
Since your test is now able to detect the platform, you can add a logic in KEYBOARD_KEY const based on the detected platform:
const os = require('os');
const platform = os.platform();
export const KEYBOARD_KEY = {
A: 'a',
ALT: 'Alt',
ARROW_DOWN: 'ArrowDown',
B: 'b',
C: 'c',
CONTROL: platform === 'darwin' ? 'Meta' : 'Control',
D: 'd',
// ...
};
await page.keyboard.press(`${KEYBOARD_KEY.CONTROL}+${KEYBOARD_KEY.C}`);
If this code is executed on the Darwin (MacOS) platform, the Meta+C will be pressed and Control+C will be pressed in other cases (Windows or Linux).
Keyboard.type()
This Playwright method sends a keyDown
, keyPress
/input
, and keyUp
event for each character in the text.
await page.keyboard.type('Hello SDEThub.com');
Keyboard.type() also supports delay to type text with user-like speed:
await page.keyboard.type('Hello SDEThub.com', { delay: 100 });
In most cases, I suggest using locator.fill() and locator.pressSequentially() instead. Please, read the appropriate guide here:
Keyboard modifiers, keyboard.up(), and keyboard.down()
One of the most popular keyboard modifiers is SHIFT. It is really simple to use it in Playwright using the keyboard.down() and the keyboard.up() methods combination.
export const KEYBOARD_KEY = {
A: 'a',
ALT: 'Alt',
ARROW_DOWN: 'ArrowDown',
B: 'b',
C: 'c',
CONTROL: 'Control',
D: 'd',
SHIFT: 'Shift',
// ...
};
await page.keyboard.down(KEYBOARD_KEY.SHIFT);
await page.locator('//*[@id = "name"]').fill('Hello SDEThub.com');
await page.keyboard.up(KEYBOARD_KEY.SHIFT);
Don’t forget to release the SHIFT button using the keyboard.up() method.
One thought on “How To Control a Keyboard in Playwright”