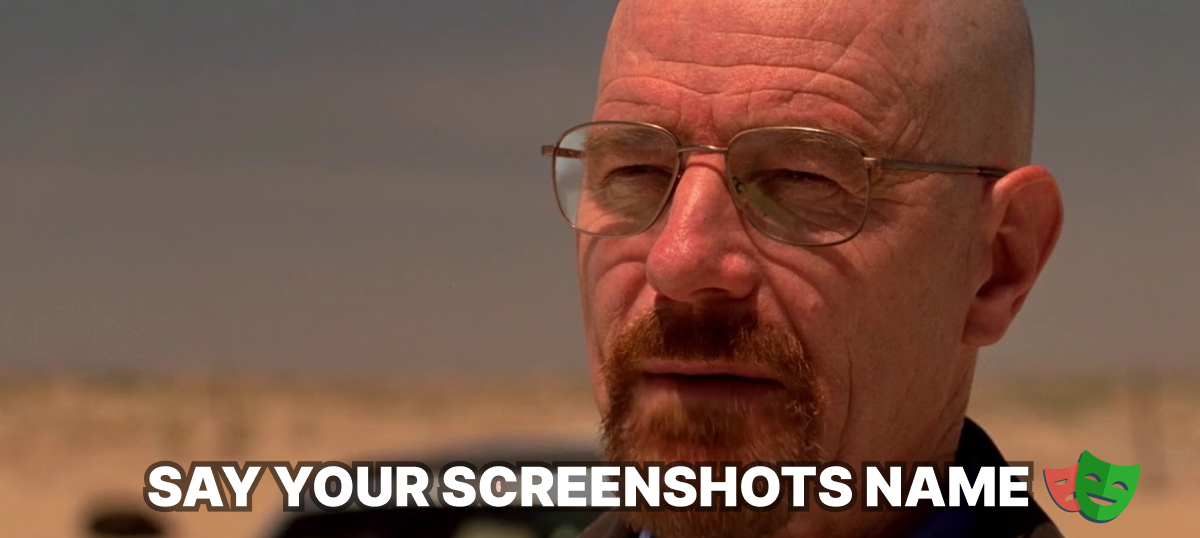
Using screenshots is a crucial part of visual testing in Playwright. When you have a lot of tests and test files in your testing project, keeping your gold screenshots in order is essential.
One of the popular questions I got from my colleagues is, “Can I somehow change the directory for saving screenshots in Playwright?”. To answer it, I’ll explain the built-in screenshot path template structure in Playwright and then show you how to change the screenshot path and include helpful details in filenames using the snapshotPathTemplate configuration option.
Default screenshots directory in Playwright
By default, Playwright wants you to keep screenshot files in a directory whose name includes your test file name. For instance, if you assert the screenshot in file /tests/example.spec.ts, your gold screenshot files should be stored in the /tests/example.spec.ts-snapshots directory.
The default file name should also include the project and the platform: example-chromium-darwin.png
Keep in mind that you don’t need to include the project and the platform in the test code:
await expect(page).toHaveScreenshot('example.png')
This is because the project and platform are dynamic and depend on the environment you are running the tests. If you have multiple projects, i.e., Chromium and Firefox, your test code will be the same (‘example.png’), but you need two different files with different projects in their names accordingly:
example-chromium-darwin.png and example-firefox-darwin.png.
Playwright screenshot path settings
Sometimes, the default approach to file naming does not fit your project structure. However, you can modify it using the playwright.config.ts configuration file you can find in the root directory of your Playwright test project.
All test screenshot path properties can be set in the snapshotPathTemplate option. This option configures a template controlling the location of snapshots generated by pageAssertions.toHaveScreenshot(name[, options]) and snapshotAssertions.toMatchSnapshot(name[, options])
The value might include some “tokens” that will be replaced with actual values during test execution.
For example, the default example.spec.ts-snapshots/example-chromium-darwin.png naming is a result of using the token structure like this:
snapshotPathTemplate: '{testDir}/{testFilePath}-snapshots/{arg}-{projectName}-{platform}{ext}'
In this example, multiple default tokens are used:
testDir: Default project’s testConfig.testDir.
testFilePath: Relative path from testDir to test file.
arg: Relative snapshot path without extension. These come from the arguments passed to the toHaveScreenshot() and toMatchSnapshot() calls; if called without arguments, this will be an auto-generated snapshot name.
projectName: Project’s file-system-sanitized name, if any.
platform: The value of process.platform.
ext: snapshot extension (with dots).
Change the default screenshot directory.
The most common change you could want to make in a test project is a different or separate directory, which doesn’t depend on the test file. It is a pretty simple task:snapshotPathTemplate:
snapshotPathTemplate: 'snapshots/{arg}-{projectName}-{platform}{ext}'
By using such a configuration, your screenshots have to be stored in the project root /snapshots directory (/snapshots/example-chromium-darwin.png)
Exclude the platform and project name.
Suppose you run your tests on only one platform or project, or your goal is pixel-perfect on every platform and browser. In that, you can also exclude appropriate tokens from the snapshotPathTemplate option:
snapshotPathTemplate: 'snapshots/{arg}{ext}'
The result of such a configuration will be /snapshots/example.png
Add Platform and Browser name-based structure
You can also introduce different file structures based on platform and project by using / separators:
snapshotPathTemplate:'snapshots/{projectName}/{platform}/{arg}{ext}'
By providing this configuration, your screenshot will stored in the platform/project structure: snapshots/chromium/darwin/example.png
Or add the test file name in:
snapshotPathTemplate: 'snapshots/{projectName}/{testFilePath}-{arg}{ext}'
In this case, your files should be stored in /snapshots/chromium/example.spec.ts-example.png
Include the test title.
Sometimes, if you want to include the test title in the screenshot name, you can use the {testName} token. Let’s check some example test:
test('TC01 Open LinkedIn URL @smoke', async ({page}) => {
await page.goto("https://www.linkedin.com/in/truuts");
await expect(page).toHaveScreenshot('example.png')
});
To include the test title in a snapshot path template, use the testName token.
testName: File-system-sanitized test title, including parent describes but excluding file name.
snapshotPathTemplate: 'snapshots/{testName}/{projectName}/{arg}{ext}'
The resulting path will be: /snapshots/TC01-Open-LinkedIn-URL-smoke/chromium/example.png
I hope this tutorial helps you to structure your screenshots in your way and optimize your test project. Happy testing!